The Cryptographic Foundation: Understanding Elliptic Curves in VRF-AD Implementation

Kona Siva Naga Malleswara Rao
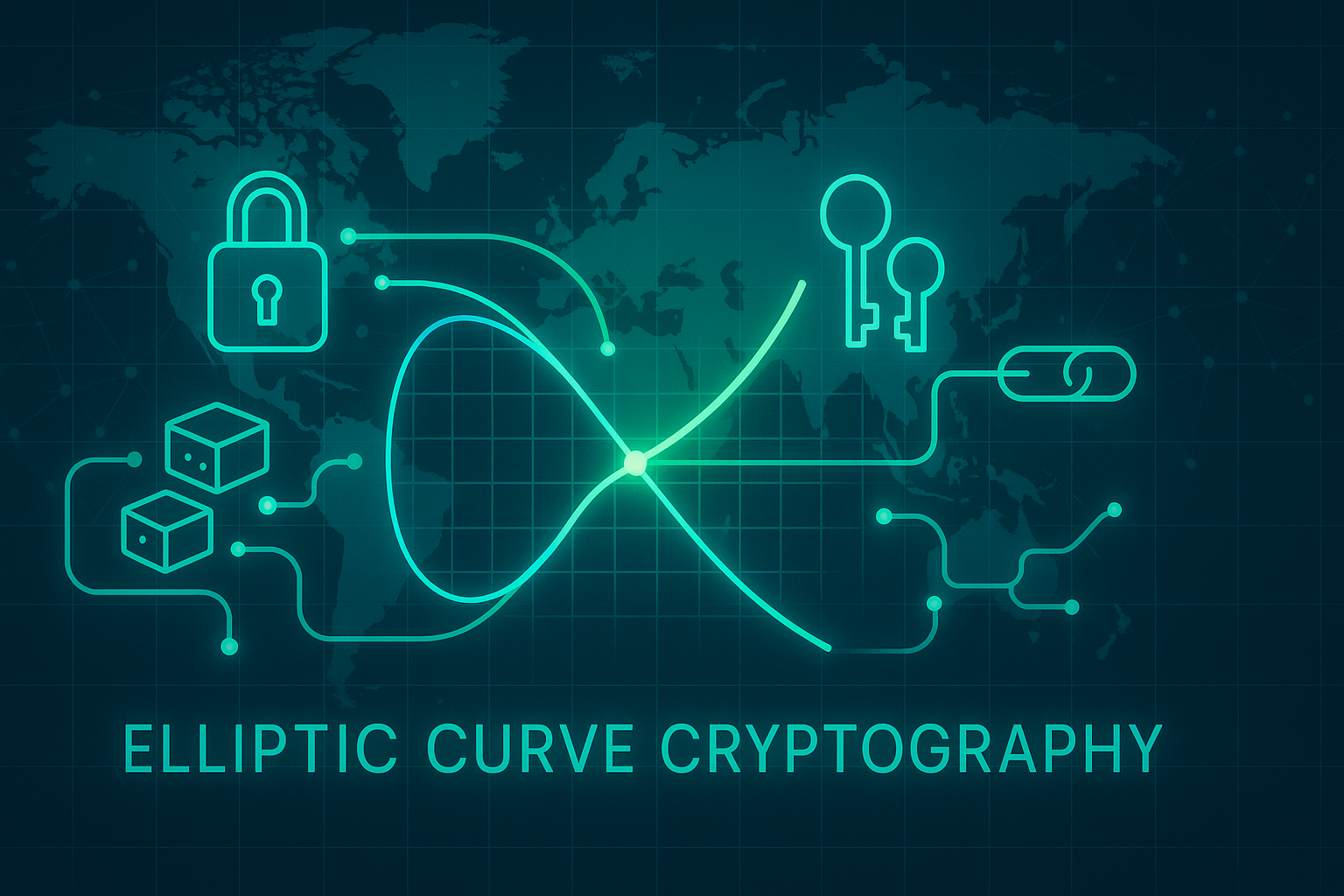
Introduction#
In our previous article, we explored how Verifiable Random Functions with Additional Data (VRF-AD) provide crucial security guarantees against replay attacks and other vulnerabilities in blockchain systems. Today, we dive deeper into the mathematical structures that make these cryptographic wonders possible: Elliptic Curves.
For many developers implementing cryptographic protocols, elliptic curves remain a mysterious black box—powerful but opaque. Yet understanding these mathematical objects is essential for making informed implementation decisions, especially when optimizing for performance, security, and gas efficiency in blockchain applications.
This article will demystify elliptic curves and explain why they're fundamental to VRF-AD implementations. We'll explore how different curve properties directly impact the security, efficiency, and implementation complexity of your VRF-AD system.
What Are Elliptic Curves?#
Despite their name, elliptic curves aren't ellipses. They're algebraic curves defined by an equation of the form:
y² = x³ + ax + b
Where a and b are constants that determine the specific curve. This seemingly simple equation creates a smooth, continuous curve with properties that make it ideal for cryptography.
The magical property of elliptic curves is that points on the curve form a mathematical group when combined with a "point at infinity" (denoted as O). This means:
- We can define a point addition operation (P + Q = R) where R is also a point on the curve
- Every point has an inverse (-P) such that P + (-P) = O
- The point addition is associative: (P + Q) + R = P + (Q + R)
These properties allow us to define scalar multiplication (multiplying a point by an integer k):
kP = P + P + ... + P (k times)
This scalar multiplication operation is the cornerstone of elliptic curve cryptography and directly enables VRF-AD functionality.
The Discrete Logarithm Problem: The Security Foundation#
What makes elliptic curves useful for cryptography is the computational asymmetry of the discrete logarithm problem:
- Given points P and Q = kP, finding k is computationally infeasible for well-chosen curves and large values of k
- However, computing Q = kP for a known k is efficient
This asymmetry is the foundation of elliptic curve cryptography (ECC) and directly enables the security properties of VRF-AD:
- Uniqueness: Only the holder of the private key k can compute kP
- Verifiability: Others can verify that a point Q equals kP without knowing k
- Unpredictability: Without knowing k, the output appears random
Elliptic Curves in VRF-AD: A Concrete Example#
Let's examine how elliptic curves enable each component of a VRF-AD system:
1. Key Generation#
VRF-AD begins with a key pair:
- Private key: A randomly selected scalar k
- Public key: The point K = kG, where G is a fixed generator point on the curve
# Key generation with elliptic curves
def generate_key_pair():
# Select a random scalar within the curve's order
private_key = random_scalar(curve_order)
# Compute the public key point through scalar multiplication
public_key = scalar_multiply(private_key, G)
return private_key, public_key
This operation depends on the security of the discrete logarithm problem—deriving k from K = kG should be computationally infeasible.
2. Input Processing#
VRF-AD requires mapping arbitrary inputs (α) and additional data (AD) to points on the elliptic curve:
# Map input and additional data to a curve point
def hash_to_curve(alpha, additional_data):
# Combine input and additional data
combined = hash_function(alpha + additional_data)
# Map the hash to a valid curve point
return map_to_curve(combined)
The map_to_curve
function is non-trivial and requires careful implementation to ensure security and efficiency. Different curves support different mapping techniques, which significantly impacts performance.
3. VRF Output Generation#
The core VRF operation uses the private key to create a deterministic but unpredictable output:
# Generate VRF output using elliptic curve scalar multiplication
def vrf_output(private_key, alpha, additional_data):
# Map input to curve point
h = hash_to_curve(alpha, additional_data)
# Core VRF operation: scalar multiplication
gamma = scalar_multiply(private_key, h)
# Convert point to output bytes
return point_to_bytes(gamma)
The security of this operation depends on the hardness of the elliptic curve discrete logarithm problem. Without knowing the private key, an attacker cannot compute the correct gamma.
4. Proof Generation#
VRF-AD requires creating a proof that can be verified without revealing the private key:
# Generate VRF proof
def generate_proof(private_key, alpha, additional_data):
# Map input to curve point
h = hash_to_curve(alpha, additional_data)
# Compute gamma
gamma = scalar_multiply(private_key, h)
# Create proof using a zero-knowledge proof system
# (typically a Schnorr-like signature)
r = random_scalar(curve_order)
commitment = scalar_multiply(r, G)
challenge = hash_points(G, public_key, h, gamma, commitment, additional_data)
response = (r + challenge * private_key) % curve_order
return gamma, (challenge, response)
This proof generation relies on the algebraic properties of the elliptic curve group.
5. Verification#
The verification process uses elliptic curve operations to check the proof:
# Verify VRF proof
def verify(public_key, alpha, additional_data, gamma, proof):
# Map input to curve point
h = hash_to_curve(alpha, additional_data)
# Extract proof components
challenge, response = proof
# Verify using elliptic curve operations
left = scalar_multiply(response, G)
right = point_add(scalar_multiply(challenge, public_key),
scalar_multiply(response, G))
# Check if the verification equation holds
computed_challenge = hash_points(G, public_key, h, gamma, right, additional_data)
return challenge == computed_challenge
The security of this verification depends on the properties of the elliptic curve group, including the discrete logarithm problem.
Critical Elliptic Curve Properties for VRF-AD#
When implementing VRF-AD, several properties of elliptic curves directly impact security and performance:
1. Order and Cofactor#
The order of a curve (n) is the number of points on the curve. For security, we want:
- n to be large (typically 256 bits or more)
- n to have a large prime factor
- The cofactor (h = #E/n) to be small, ideally 1
The cofactor particularly affects the security of mapping functions used in VRF-AD.
2. Resistance to Known Attacks#
Different curves offer varying levels of resistance to:
- Pollard's rho algorithm
- MOV attack
- Invalid curve attacks
- Small subgroup attacks
These security properties directly impact the security guarantees of your VRF-AD implementation.
3. Efficiency of Operations#
The curve shape and parameters affect computational efficiency:
- Addition formulas complexity
- Scalar multiplication algorithms
- Encoding/decoding overhead
For VRF-AD implementations, these efficiency considerations directly impact:
- Proof generation time
- Verification costs (especially important for on-chain verification)
- Gas costs in blockchain implementations
Common Elliptic Curves and Their Impact on VRF-AD#
Different standard curves offer different tradeoffs for VRF-AD implementations:
1. NIST Curves (P-256, P-384)#
- Advantages: Widely implemented, regulatory compliance
- Disadvantages: Less efficient formulas, some theoretical concerns
- Impact on VRF-AD: Higher computational overhead, but easier adoption in regulated environments
2. Curve25519 (Edwards25519)#
- Advantages: Efficient formulas, side-channel resistance
- Disadvantages: Less hardware acceleration
- Impact on VRF-AD: Faster proof generation, better side-channel security
3. secp256k1 (Bitcoin curve)#
- Advantages: Widespread blockchain adoption, hardware acceleration
- Disadvantages: Not as optimized for zero-knowledge proofs
- Impact on VRF-AD: Good performance but less efficient for advanced proof systems
4. BLS12-381#
- Advantages: Pairing-friendly, excellent for zero-knowledge proofs
- Disadvantages: More complex, higher overhead for basic operations
- Impact on VRF-AD: Excellent when combining VRF-AD with advanced zero-knowledge systems
5. Bandersnatch#
- Advantages: Optimized for zero-knowledge proofs, supports efficient algorithms
- Disadvantages: Newer, less widely implemented
- Impact on VRF-AD: Superior performance for advanced VRF-AD implementations
Implementation Considerations#
Your choice of elliptic curve directly impacts several crucial aspects of VRF-AD implementation:
1. Encoding Methods (hash_to_curve)#
Different curves support different methods for mapping inputs to curve points:
- Try-and-increment: Simple but non-constant time
- Elligator: Efficient for certain curve types
The efficiency and security of these mapping methods vary significantly between curves, directly affecting VRF-AD performance.
# Example: Different hash-to-curve implementations
def try_and_increment(input_bytes):
# Simple but non-constant time (vulnerable to timing attacks)
counter = 0
while True:
attempt = hash_function(input_bytes + counter.to_bytes(4, 'big'))
if is_valid_x_coordinate(attempt):
return recover_point(attempt)
counter += 1
2. Scalar Multiplication Optimizations#
Scalar multiplication is the most computationally intensive operation in VRF-AD. Different curves support different optimization techniques:
- Window methods: Process multiple bits at once
- GLV/GLS method: Use endomorphisms to speed up computation
- Fixed-base optimization: For repeatedly using the same base point
Some curves like Bandersnatch are specifically designed to enable these optimizations, dramatically improving VRF-AD performance.
3. Side-Channel Resistance#
Different curves and implementations offer varying degrees of protection against:
- Timing attacks: Variations in execution time reveal information
- Power analysis: Energy consumption patterns reveal information
- Cache attacks: Memory access patterns reveal information
These side-channel considerations are vital for secure VRF-AD implementations, especially in untrusted environments.
4. Gas Efficiency: The Blockchain Perspective#
For blockchain applications, gas efficiency is paramount. Your choice of elliptic curve directly impacts on-chain verification costs.
Conclusion#
Elliptic curves are not just an implementation detail of VRF-AD—they are the fundamental mathematical structure that enables its security and efficiency. Understanding the properties and tradeoffs of different curves is essential for building optimal VRF-AD systems.
In our next article, we'll dive deeper into specific optimizations for VRF-AD implementation, focusing on how the Bandersnatch curve offers significant advantages for advanced cryptographic applications. We'll explore its unique properties and show how they translate to performance improvements, lower gas costs, and enhanced security in real-world VRF-AD deployments.
By building this foundation in elliptic curve understanding, you'll be better equipped to make informed decisions about your VRF-AD implementation, optimizing for security, performance, and cost-effectiveness.
Want to learn more about our work with VRF-AD and cryptographic primitives? Visit ChainScore Finance or reach out to our team for collaboration opportunities.

About Kona Siva Naga Malleswara Rao
Continue Reading
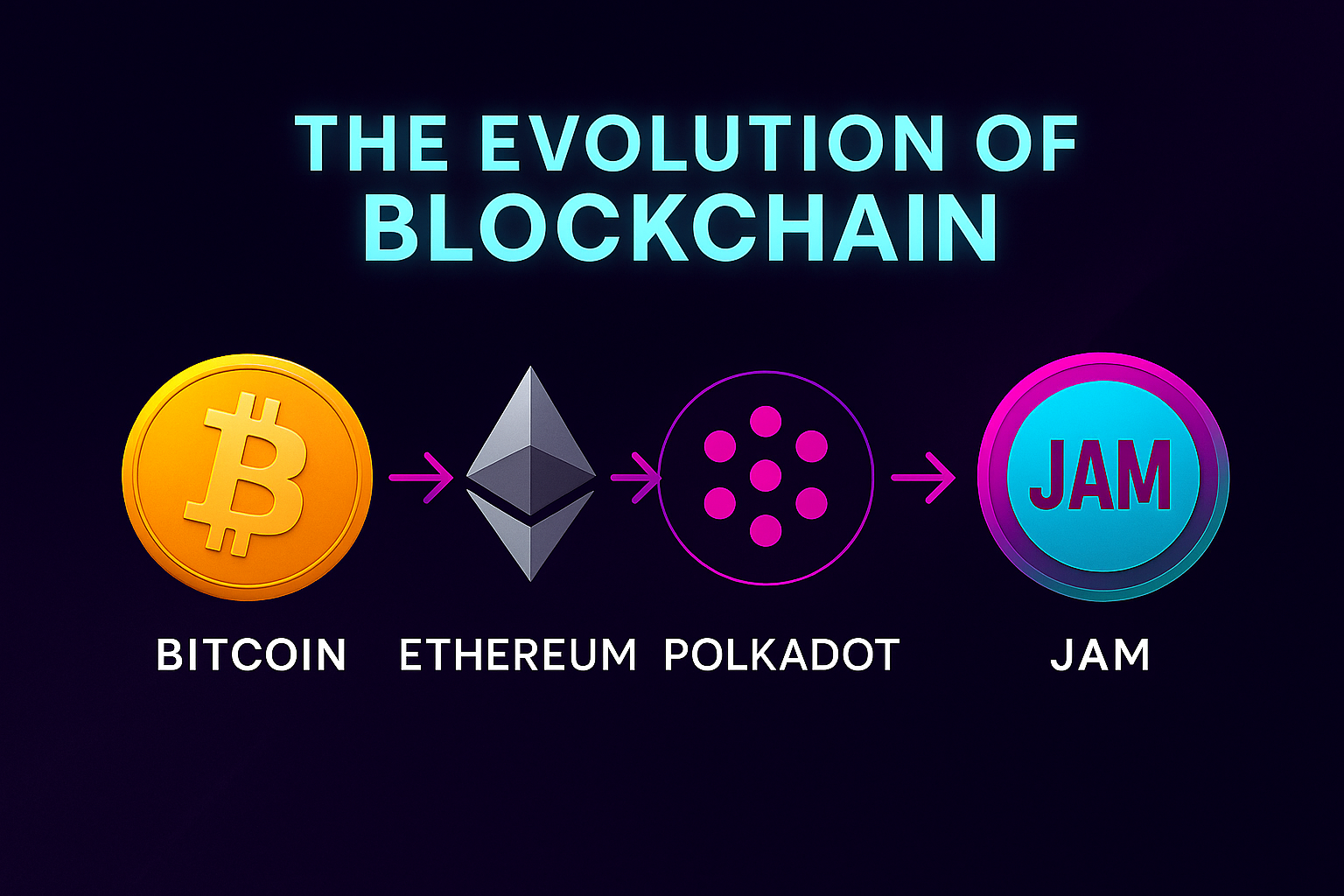
The Evolution of Blockchain: From Bitcoin to JAM
Blockchain evolved from Bitcoin’s simple ledger to JAM’s rollup-native model, reshaping the internet and pushing the boundaries of security, decentralization, and scalability.

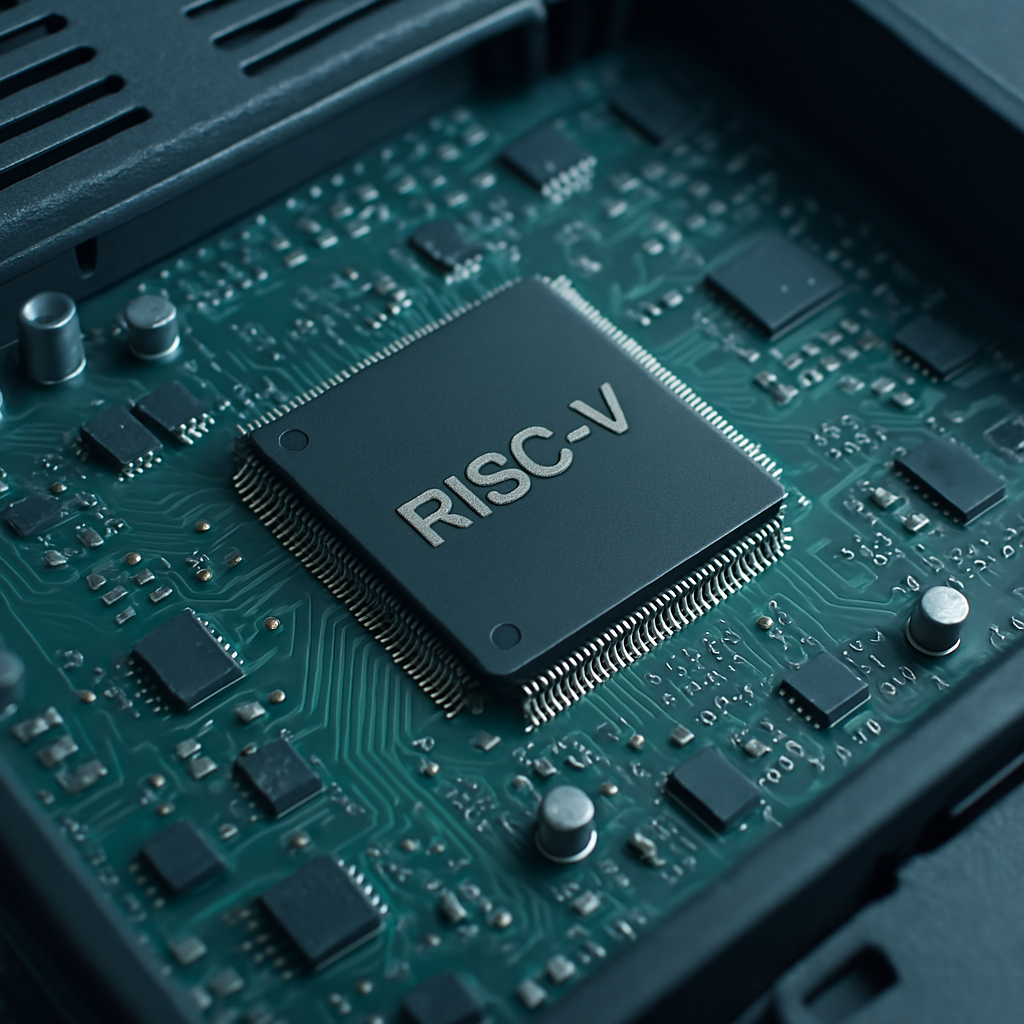
From Code to CPU: How JAM Ensures Consistent Execution on RISC-V Hardware
Explore how JAM ensures consistent execution on RISC-V hardware, from memory limits to gas constraints, and how it bridges virtual and physical environments
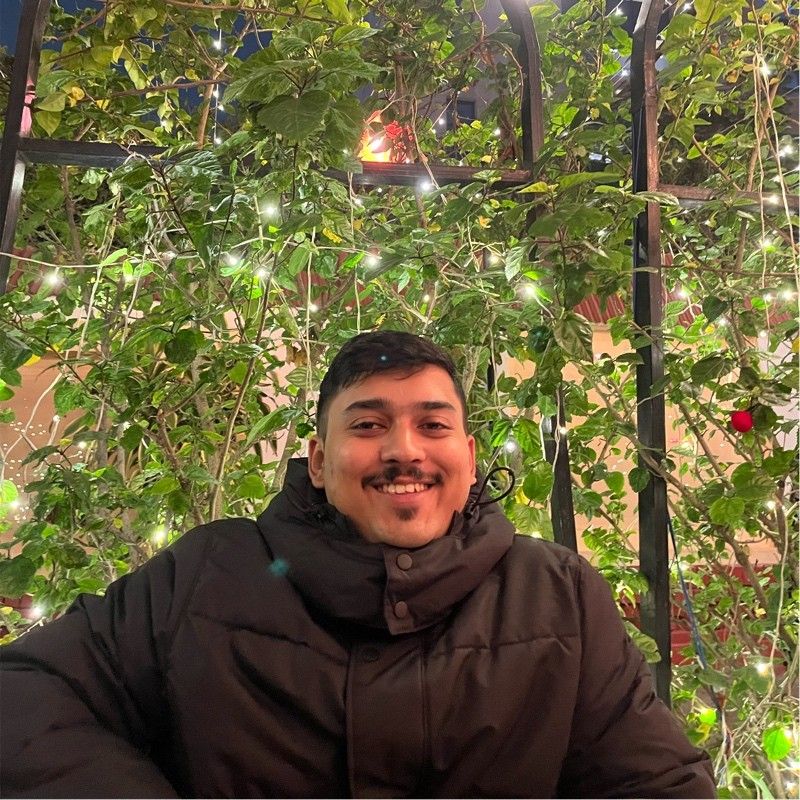